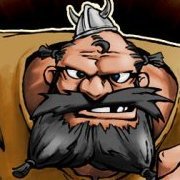
Control all your Powered Up & Power Function (SBrick) devices with a single software
By
Cosmik42, in LEGO Train Tech
-
Recently Browsing 0 members
No registered users viewing this page.
By
Cosmik42, in LEGO Train Tech
No registered users viewing this page.