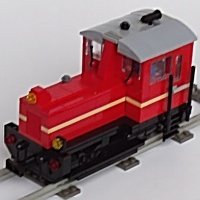
„Libraries“ for Pybricks
By
Lok24, in LEGO Technic, Mindstorms, Model Team and Scale Modeling
-
Recently Browsing 0 members
No registered users viewing this page.
By
Lok24, in LEGO Technic, Mindstorms, Model Team and Scale Modeling
No registered users viewing this page.